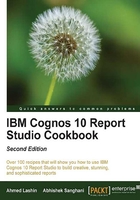
Manipulating the Date Time control
There is a report that allows users to filter on Shipment Date Time using the Date Time control. By default, Cognos selects the current date and midnight as the date and time.
Report Studio allows you to override this with another static default value. However, a business will usually run the report for the end of the previous business day (5 pm).
In this recipe, we will learn how to change the default date and time for a Date Time control to the end of the previous business day.
Getting ready
Create a dummy report that shows sales quantity by shipment day. Define a filter on shipment day.
How to do it...
In this recipe, we want to change the default date and time for a Date Time control to the end of the previous business day using JavaScript. To do this, perform the following steps:
- We will start by adding a Date Time control to the report. For that, add a new prompt page.
- From Toolbox, drag Date & Time Prompt onto the prompt page. Connect it to the Shipment Day filter using an appropriate parameter in the prompt wizard.
- Now select the prompt and set its Name property to ShipmentDate as shown in the following screenshot:
- Now add an HTML item to the prompt footer after the Finish button. Define it as follows:
<script> function subtractDay () { var dtToday = new Date(); var dtYesterday = new Date( dtToday - 86400000 ); // NOTE 86400000 = 24 hours * 60 (minutes per hour) * 60 (seconds per minute) * 1000 milliseconds per second) var strYesterday = [dtYesterday.getUTCFullYear(), dtYesterday.getMonth()+1, dtYesterday.getDate()].join("-"); return strYesterday; } function subtractTime () { var Time = "17:00:00.000"; return Time; } pickerControlShipmentDate.setValue( subtractDay() ); timePickerShipmentDate.setValue( subtractTime() ); </script>
- Run the report to test it. You will see that the value of the Date Time control is set to the previous day, which is 5 pm by default.
How it works...
Here we use standard JavaScript functions to work out the date of the previous day. Please note that this date is computed based on the system date on the user's machine.
Then, we apply this date to the Date Time control using a pickerControl<name>
object. Also, we set the time to 5 pm using the setValue
function of the timePicker<name>
object.
You can similarly do more date and string manipulations to find First
of
Month
, Last
of
Month
, and so on. I found the following script on the Internet for generating commonly used dates:
<script language="JavaScript" runat="SERVER"> var today = new Date(); var thisYear = today.getYear(); var thisMonth = today.getMonth(); var thisDay = today.getDate(); function rw(s1, s2) { Response.Write("<tr><td>"+s1+"</td><td>"+s2+"</td></tr>"); } Response.Write("<table border='1'>"); rw("Today:", today.toDateString()); //Years var fdly = new Date(thisYear - 1, 0, 1); rw("First day of last year:", fdly.toDateString()); var ldly = new Date(thisYear, 0, 0); rw("Last day of last year:", ldly.toDateString()); var fdty = new Date(thisYear, 0, 1); rw("First day of this year:", fdty.toDateString()); var ldty = new Date(thisYear + 1, 0, 0); rw("Last day of this year:", ldty.toDateString()); var fdny = new Date(thisYear + 1, 0 ,1); rw("First day of next year:", fdny.toDateString()); var ldny = new Date(thisYear + 2, 0, 0); rw("Last day of next year:", ldny.toDateString()); //Months var fdlm = new Date(thisYear, thisMonth - 1 ,1); rw("First day of last month:", fdlm.toDateString()); var ldlm = new Date(thisYear, thisMonth, 0); rw("Last day of last month:", ldlm.toDateString()); rw("Number of days in last month:", ldlm.getDate()); var fdtm = new Date(thisYear, thisMonth, 1); rw("First day of this month:", fdtm.toDateString()); var ldtm = new Date(thisYear, thisMonth + 1, 0); rw("Last day of this month:", ldtm.toDateString()); rw("Number of days in this month:", ldtm.getDate()) var fdnm = new Date(thisYear, thisMonth + 1, 1); rw("First day of next month:", fdnm.toDateString()); var ldnm = new Date(thisYear, thisMonth + 2, 0); rw("Last day of next month:", ldnm.toDateString()); rw("Number of days in next month:", ldnm.getDate()); Response.Write("</table>"); </script>
There's more...
You can write more sophisticated functions to work out the previous working day instead of just the previous day.
You can mix this technique with other recipes in this chapter to tie the selection event with the button click or radio buttons; that is, a particular date/time can be selected when a user clicks on the button or selects a radio button.