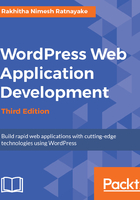
Activating system users
Once the user clicks on the activation link, redirection will be made to the /user/activate URL of the application. So, we need to modify our controller with a new case for activation, as shown in the following code:
case 'activate':
do_action( 'wpwaf_before_activate_user' );
$wpwaf->registration->activate_user();
do_action( 'wpwaf_after_activate_user' );
break;
Here, we have two actions, one before the activation function and one after the activation function. These actions can be used to alter the activation process as we did with displaying the registration form. We can use the wpwa_before_activate_user action to check certain conditions on the activation URL and then allow/deny the user to activate the account. Similarly, we can use the wpwa_after_activate_user action when we need to add additional parameters to the user in the database on successful activation.
Now let's move on to the main part of completing user activation. We have to add a new function called activate_user to the WPWAF_Registration class. Let's look at the implementation of the activate_user function:
public function activate_user() {
$activation_code = isset( $_GET['wpwaf_activation_code'] ) ? sanitize_text_field($_GET['wpwaf_activation_code']) : '';
$message = '';
$user_query = new WP_User_Query( array(
'meta_key' => 'wpwaf_activation_code',
'meta_value' => $activation_code ));
$users = $user_query->get_results();
if ( !empty($users) ) {
$user_id = $users[0]->ID;
update_user_meta( $user_id, 'wpwa_activation_status', 'active' );
$message = __('Account activated successfully.','wpwaf');
} else {
$message = __('Invalid Activation Code','wpwaf');
}
include WPWAF_PLUGIN_DIR . 'templates/info-template.php';
exit;
}
We will get the activation code from the link and query the database to find a matching entry. If no records are found, we set the message as activation failed, or else we can update the activation status of the matching user to activate the account. Upon activation, the user will be given a message using the info-template.php template, which consists of a very basic template like the following one:
<?php get_header(); ?>
<div id='wpwaf_custom_panel'>
<div id='wpwaf_info_message'>
<?php echo $message; ?>
</div>
</div>
<?php get_footer(); ?>
Once the user visits the activation page on the /user/activation URL, information will be given to the user, as illustrated in the following screenshot:

We have successfully created and activated a new user. The final task of this process is to authenticate and log the user into the system. Let's see how we can create the login functionality.