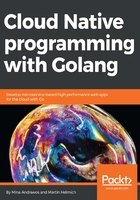
MongoDB
If you are not already familiar with the MongoDB NoSQL database engine, this section will be very useful for you.
MongoDB is a NoSQL document store database engine. The two keywords to understand MongoDB are NoSQL and document store.
NoSQL is a relatively recent keyword in the software industry that is used to indicate that a database engine does not deeply rely on relational data. Relational data is the idea that there are webs of relations between different pieces of data in your database, following the relations between your data will build a full picture of what the data represents.
Take MySQL as an example of a relational database. Data gets stored in numerous tables, then, primary and foreign keys are used to define the relations between the different tables. MongoDB doesn't work this way, which is why MySQL is considered as a SQL database, whereas MongoDB is considered NoSQL.
If you are not yet familiar with Mongodb, or don’t have a local installation you can test with. Go to https://docs.mongodb.com/manual/installation/ , where you find a list of helpful links to guide through the process of installing and running the database in your operating system of choice. Generally, when installed, Mongodb provides two key binaries: mongod and mongo. The mongod command is what you need to execute, in order to run your database. Any software you then write will communicate with mongod to access Mongodb’s data. The mongo command, on the other hand, is basically a client tool you can use to test the data on Mongodb, the mongo command communicates with mongod, similarly to any application you write that accesses the database.
There are two flavors of MongoDB: the community edition, and the enterprise edition. Obviously, the enterprise edition targets larger enterprise installations, whereas the community edition is what you’d use for your testing and smaller scale deployments. Here are the links for the community edition guides covering the three major operating systems:
- For linux Mongodb installation and deployment: https://docs.mongodb.com/manual/administration/install-on-linux/
- For Windows Mongodb installation and deployment: https://docs.mongodb.com/manual/tutorial/install-mongodb-on-windows/
- For OS X Mongodb installation and deployment: https://docs.mongodb.com/manual/tutorial/install-mongodb-on-os-x/
Overall, there are three main steps you need to consider when deploying a Mongodb instance:
- Install Mongodb for your operating system, the download page can be found here: https://www.mongodb.com/download-center
- Ensure MongoDB’s key binaries are defined in your environmental path, so that you can run them from the terminal no matter what your current directory is. The key binaries are mongod and mongo. Another binary worth mentioning is mongos, which is important if you are planning to utilize clustering
- Run the mongod command with no arguments, and this will run Mongodb with all your default settings. Alternatively, you can use it with different configuration. You can either use a configuration file or just runtime parameters. You can find information about the configuration file here: https://docs.mongodb.com/manual/reference/configuration-options/#configuration-file. To start mongod with a custom configuration file, you can use the --config option, here is an example: mongod --config /etc/mongod.conf. On the other hand, for runtime parameters, you can just use --option to change an option when running mongod, for example you can type mongod --port 5454 to start mongod on a different port than the default
There are different types of NoSQL databases. One of these types is the document store database. The idea of a document store is that data gets stored in numerous document files stacked together to represent what we are trying to store. Let's take the data store needed for the event's microservice as an example. If we are using a document store in the microservice persistence layer, each event will be stored in a separate document with a unique ID. Say that we have an Opera Aida event, a Coldplay concert event, and a ballet performance event. In MongoDB, we would create a collection of documents called events, which will contain three documents—one for the Opera, one for Coldplay, and one for the ballet performance.
So, to solidify our understanding of how MongoDB would represent this data, here is a diagram of the events collection:

Events collection
Collections and documents are important concepts in MongoDB. A production MongoDB environment typically consists of multiple collections; each collection would represent a different piece of our data. So, for example, our MyEvents application consists of a number of microservices, each microservice cares about a distinctive piece of data. The bookings microservice would store data in a bookings collection, and the events microservices would store data in an events collection. We'd also need the user's data to be stored separately in order to manage the users of our applications independently. Here is what this would end up looking like:

Our MongoDB database
You can download this file from https://www.packtpub.com/sites/default/files/downloads/CloudNativeprogrammingwithGolang_ColorImages.pdf.
The code bundle for the book is also hosted on GitHub at https://github.com/PacktPublishing/Cloud-Native-Programming-with-Golang.
Since we have focused so far on the events microservice as a showcase on how to build a microservice, let's pe deeper into the events collection, which would be used by the event's microservice:

Events collection
Each document in the events collection needs to include all the information necessary to represent a single event. Here's how an event document should look like:

If you haven't noticed already, the preceding JSON document is the same as the HTTP body document that we presented as an example of what the add event API HTTP POST request body looks like.
In order to write software that can work with this data, we need to create models. Models are basically data structures containing fields that match the data we are expecting from the database. In the case of Go, we'd use struct types to create our models. Here is what a model for an event should look like:
type Event struct {
ID bson.ObjectId `bson:"_id"`
Name string
Duration int
StartDate int64
EndDate int64
Location Location
}
type Location struct {
Name string
Address string
Country string
OpenTime int
CloseTime int
Halls []Hall
}
type Hall struct {
Name string `json:"name"`
Location string `json:"location,omitempty"`
Capacity int `json:"capacity"`
}
The Event struct is the data structure or model for our event document. It contains the ID, event name, event duration, event start date, event end date, and event location. Since the event location needs to hold more information than just a single field, we will create a struct type called location to model a location. The Location struct type contains the location's name, address, country, open time and close time, and the halls in that area. A hall is basically the room inside the location where the event is taking place.
So, for example, Mountain View, Opera house in downtown Mountain View would be the location, whereas the silicon valley room on the east side would be the hall.
In turn, the hall cannot be represented by a single field since we need to know its name, its location in the building (south-east, west, and so forth), and its capacity (the number of people it can host).
The bson.ObjectId type in the event struct is a special type that represents MongoDB document ID. The bson package can be found in the mgo adapter, which is the Go third part framework of choice to communicate with MongoDB. The bson.ObjectId type also provides some helpful methods that we can use later in our code to verify the validity of the ID.
Before we start covering mgo, let's take a moment to explain what bson means. bson is a data format used by MongoDB to represent data in stored documents. It could be simply considered as binary JSON because it is a binary-encoded serialization of JSON-like documents. The specification can be found at: http://bsonspec.org/.
Now, let's cover mgo.