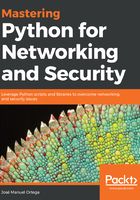
Threading module
In addition to the thread module, we have another approach to using the threading module. The threading module relies on the thread module to provide us a higher level, more complete, and object-oriented API. The threading module is based slightly on the Java threads model.
The threading module contains a Thread class that we must extend to create our own threads of execution. The run method will contain the code that we want the thread to execute. If we want to specify our own constructor, it must call threading. Thread .__ init __ (self) to initialize the object correctly.
Before creating a new thread in Python, we review the Python Thread class init method constructor and see what parameters we need to pass in:
# Python Thread class Constructor
def __init__(self, group=None, target=None, name=None, args=(), kwargs=None, verbose=None):
The Thread class constructor accepts five arguments as parameters:
- group: A special parameter that is reserved for future extensions.
- target: The callable object to be invoked by the run method().
- name: Our thread's name.
- args: Argument tuple for target invocation.
- kwargs: Dictionary keyword argument to invoke the base class constructor.
We can get more information about the init() method if we invoke the help(threading) command in a Python interpreter console:
Let’s create a simple script that we’ll then use to create our first thread:
You can find the following code in the threading_init.py file in threads subfolder:
import threading
def myTask():
print("Hello World: {}".format(threading.current_thread()))
# We create our first thread and pass in our myTask function
myFirstThread = threading.Thread(target=myTask)
# We start out thread
myFirstThread.start()
In order for the thread to start executing its code, it is enough to create an instance of the class that we just defined and call its start method. The code of the main thread and that of the one that we have just created will be executed concurrently.
We have to instantiate a Thread object and invoke the start() method. Run is our logic that we wish to *run* in parallel inside each of our threads, so we can use the run() method to launch a new thread. This method will contain the code that we want to execute in parallel.
In this script, we are creating four threads.
You can find the following code in the threading_example.py file in threads subfolder:
import threading
class MyThread(threading.Thread):
def __init__ (self, message):
threading.Thread.__init__(self)
self.message = message
def run(self):
print self.message
threads = []
for num in range(0, 5):
thread = MyThread("I am the "+str(num)+" thread")
thread.name = num
thread.start()
We can also use the thread.join() method to wait until the thread terminates. The join method is used so that the thread that executes the call is blocked until the thread on which it is called ends. In this case, it is used so that the main thread does not finish its execution before the children, which could result in some platforms in the termination of the children before finishing its execution. The join method can take a floating point number as a parameter, indicating the maximum number of seconds to wait.
You can find the following code in the threading_join.py file in threads subfolder:
import threading
class thread_message(threading.Thread):
def __init__ (self, message):
threading.Thread.__init__(self)
self.message = message
def run(self):
print self.message
threads = []
for num in range(0, 10):
thread = thread_message("I am the "+str(num)+" thread")
thread.start()
threads.append(thread)
# wait for all threads to complete by entering them
for thread in threads:
thread.join()